In a small village, a curious boy named Leo discovered a mysterious old clock in his grandfather’s attic. Each hour, the clock chimed, and with each chime, a tiny door opened, revealing a new scene: a bustling market, a serene forest, a starry night. Intrigued, Leo realized that every hour, the clock repeated the same scenes, like a loop in time. He spent days watching, learning the stories behind each moment. The clock taught him that life, much like its chimes, often circles back, offering us chances to revisit and reflect.
Table of Contents
- Understanding the Concept of Loops in Programming
- Exploring Real-World Examples of Loops in Action
- Analyzing the Benefits of Using Loops for Efficiency
- Best Practices for Implementing Loops in Your Code
- Q&A
Understanding the Concept of Loops in Programming
Loops are fundamental constructs in programming that allow developers to execute a block of code multiple times without the need to rewrite it. This repetition can be controlled through various conditions, making loops incredibly versatile. For instance, a simple loop can be used to iterate through a list of items, performing the same operation on each element. This not only saves time but also enhances code readability and maintainability.
One of the most common types of loops is the for loop. This loop is particularly useful when the number of iterations is known beforehand. For example, if you want to print the numbers from 1 to 5, a for loop can succinctly handle this task:
- Initialization: Set a starting point.
- Condition: Define when the loop should stop.
- Increment/Decrement: Update the loop variable after each iteration.
In this case, the loop would look something like this in Python:
for i in range(1, 6):
print(i)
Another popular loop is the while loop, which continues to execute as long as a specified condition remains true. This type of loop is particularly useful when the number of iterations is not predetermined. For example, if you want to keep asking a user for input until they provide a valid response, a while loop can be employed:
user_input = ''
while user_input != 'exit':
user_input = input('Type "exit" to stop: ')
In this scenario, the loop will persist until the user types “exit,” demonstrating how loops can adapt to dynamic conditions and user interactions.
Exploring Real-World Examples of Loops in Action
Loops are not just a concept confined to the realm of programming; they are woven into the fabric of our daily lives. Consider the simple act of preparing breakfast. Each morning, you might follow a series of steps that repeat in a specific order: gathering ingredients, cooking, and serving. This routine can be likened to a loop, where the process is executed repeatedly until a certain condition is met—like the completion of your meal. In this way, loops help streamline our actions, making them more efficient and predictable.
Another vivid example can be found in nature. Take the life cycle of a butterfly, which can be viewed as a loop of transformation. The stages—egg, larva, pupa, and adult—repeat in a continuous cycle. Each stage is crucial, and the completion of one leads to the beginning of another. This natural loop not only illustrates the concept of repetition but also highlights the importance of each phase in achieving the final outcome. Just as in programming, where loops allow for repeated execution of code, nature employs loops to ensure the survival and evolution of species.
In the world of technology, loops are fundamental to the functioning of various devices. For instance, consider a smart thermostat that continuously monitors the temperature of a room. It operates in a loop, checking the current temperature against the desired setting. If the temperature falls below the set point, the thermostat activates the heating system. This process repeats until the desired temperature is achieved. Such loops are essential for maintaining comfort and efficiency in our homes, showcasing how programming concepts translate into practical applications.
Lastly, think about the way we learn new skills, such as playing a musical instrument. Practice often involves repeating specific exercises or pieces of music until mastery is achieved. This repetitive process can be seen as a loop, where each iteration builds upon the last. As we refine our technique, we create a feedback loop that enhances our performance. This example not only illustrates the concept of loops in a personal context but also emphasizes the role of repetition in achieving excellence, whether in music, sports, or any other discipline.
Analyzing the Benefits of Using Loops for Efficiency
Loops are a fundamental concept in programming that allow developers to execute a block of code multiple times without the need for repetitive writing. This not only enhances the readability of the code but also significantly reduces the chances of errors. By utilizing loops, programmers can streamline their workflows and focus on more complex logic rather than getting bogged down by repetitive tasks.
One of the primary advantages of using loops is their ability to handle large datasets efficiently. For instance, when processing a list of items, a loop can iterate through each element, applying the same operation without the need for separate code for each item. This leads to a more concise and maintainable codebase. Consider the following benefits:
- Reduced Code Duplication: Loops eliminate the need to write the same code multiple times, making it easier to manage and update.
- Dynamic Data Handling: Loops can adapt to varying sizes of data, allowing for flexibility in programming.
- Improved Performance: By minimizing the amount of code executed, loops can enhance the overall performance of applications.
Moreover, loops can be combined with conditional statements to create complex algorithms that can solve intricate problems efficiently. For example, a loop can be used to traverse through a collection of user inputs, applying specific conditions to filter or modify the data as needed. This capability not only saves time but also empowers developers to implement sophisticated logic with ease.
In addition to their practical benefits, loops also foster a deeper understanding of programming concepts. By working with loops, developers learn about iteration, control flow, and the importance of algorithmic thinking. This foundational knowledge is crucial for tackling more advanced programming challenges and contributes to a programmer’s overall skill set. Embracing loops as a tool for efficiency ultimately leads to better coding practices and more robust applications.
Best Practices for Implementing Loops in Your Code
When implementing loops in your code, clarity and efficiency should be your guiding principles. A well-structured loop not only enhances readability but also optimizes performance. To achieve this, consider the following strategies:
- Choose the Right Loop Type: Depending on your needs, select between for, while, or do-while loops. Each serves a unique purpose and can significantly affect the flow of your program.
- Limit Loop Scope: Keep the loop’s scope as narrow as possible. This minimizes the risk of unintended side effects and makes your code easier to debug.
- Use Descriptive Variable Names: Instead of generic names like i or j, opt for more descriptive names that convey the purpose of the variable, enhancing code readability.
Another essential practice is to avoid infinite loops, which can cause your program to hang or crash. To prevent this, always ensure that your loop has a clear exit condition. This can be achieved by:
- Defining Clear Conditions: Make sure the condition that controls the loop is well-defined and reachable.
- Implementing Break Statements: Use break statements judiciously to exit loops when certain conditions are met, providing a safety net against infinite iterations.
Performance is also a critical consideration when working with loops. To enhance efficiency, consider the following:
- Avoid Unnecessary Computations: Move calculations that do not need to be repeated outside the loop to reduce processing time.
- Utilize Built-in Functions: Whenever possible, leverage built-in functions or libraries that are optimized for performance, rather than writing your own loop from scratch.
Lastly, always prioritize code readability and maintainability. This can be achieved by:
- Commenting Your Code: Provide comments that explain the purpose of the loop and any complex logic involved, making it easier for others (or yourself in the future) to understand.
- Refactoring When Necessary: If a loop becomes too complex, consider breaking it down into smaller functions or methods to simplify the logic and improve maintainability.
Q&A
-
What is a loop in programming?
A loop is a programming construct that allows you to execute a block of code repeatedly based on a specified condition. It helps automate repetitive tasks efficiently.
-
Can you provide an example of a loop?
Sure! Here’s a simple example using a
for
loop in Python:for i in range(5): print(i)
This loop will print the numbers 0 through 4.
-
What are the types of loops?
There are several types of loops, including:
- For Loop: Iterates over a sequence (like a list or range).
- While Loop: Continues executing as long as a specified condition is true.
- Do-While Loop: Executes the block of code at least once before checking the condition.
-
Why are loops important in programming?
Loops are crucial because they:
- Reduce code redundancy by allowing repeated execution of code.
- Enhance efficiency by automating repetitive tasks.
- Facilitate complex operations, such as iterating through data structures.
In the intricate dance of programming, loops are the rhythm that keeps the melody flowing. Whether counting numbers or iterating through data, they simplify complexity. Embrace the power of loops, and let your code sing in harmony!
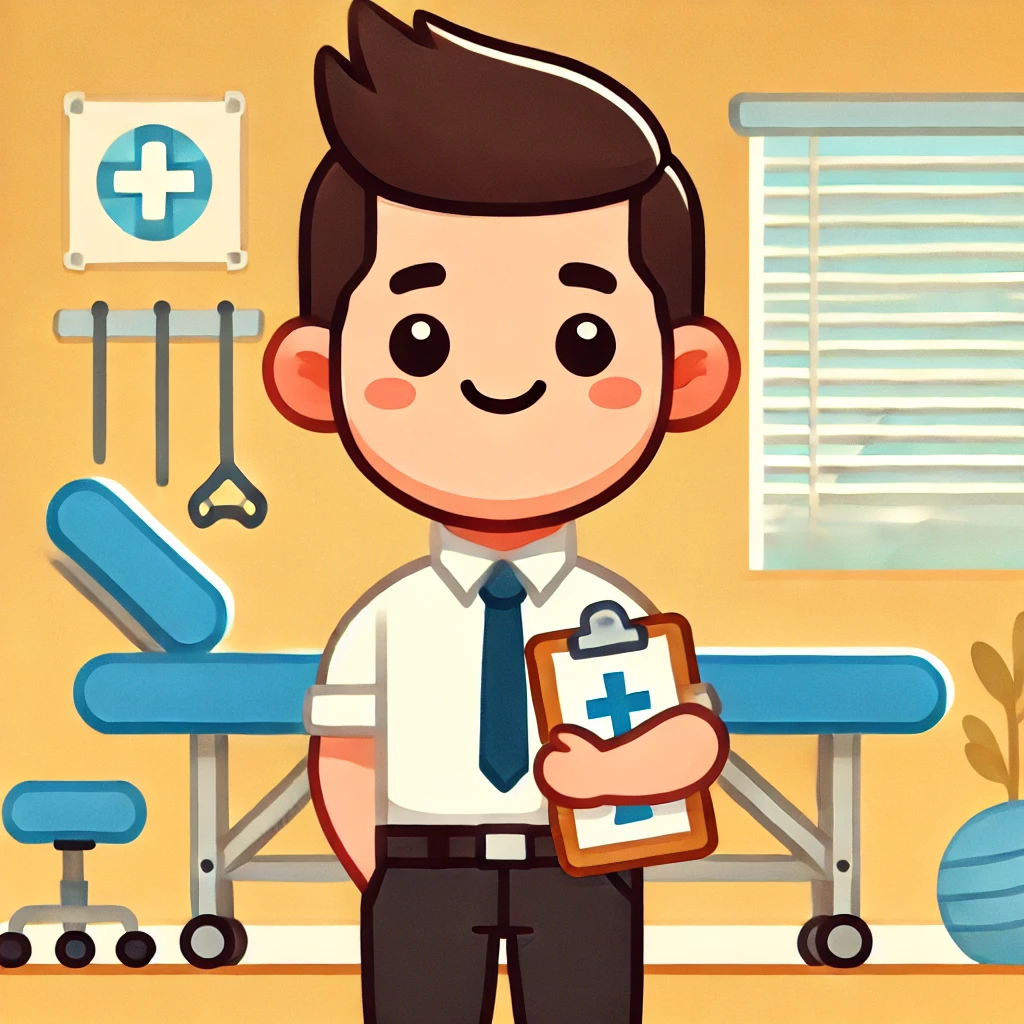
大家好,我是彼得潘,專業的手法身體治療師。我喜歡探索和研究各種主題,並透過與人工智慧的合作分享專業、實用、有趣的文章。我們定期進行人工審核,以確保內容的準確性。如果您發現文章中有任何不準確的地方,請隨時與我們聯繫,我們會及時糾正。您可以透過 [email protected] 與我們聯繫。